The previous lesson of PHP Lessons Series was about converting an image to a string and in this lesson we will learn to write text to an image using php imagettftext() function.
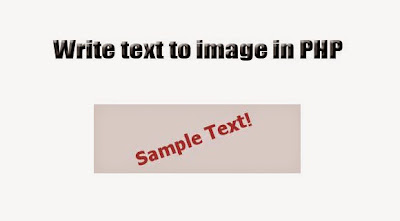
PHP imagettftext() function is used to write text to an image. TTF here stands for True Type Fonts. It means that imagettftext() function requires a (ttf) font file to write text to an image. The basic syntax of imagettftext()function is given below:
Let us use the above imagettftext() function to write a simple text to an image using php code given below:
The above php code will write the text ('Sample Text!') to an image file created using imagecreate() function. The width of the image is set to 300 and height is set to 100 pixels. The background colour allocated to the image using imagecolorallocate() function is white rgb(255,255,255) and text colour is black rgb(0,0,0). In the next step the parameters set above are passed into imagettftext() function to write text to the image. The output image of the above code should look something like this:
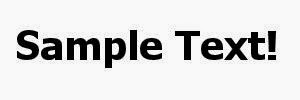
Note: Do not forget to add the font.ttf file used in the imagettftext() function above to the same directory where your php code is located. You can download TTF files here.
Playing around a bit with the parameters of imagettftext() function will produce different results.
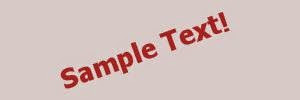
Stay tuned for more lessons...
Related Posts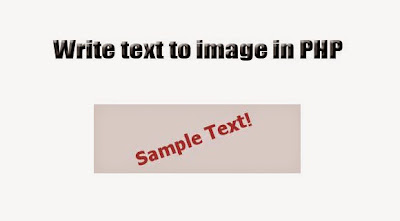
PHP imagettftext() function is used to write text to an image. TTF here stands for True Type Fonts. It means that imagettftext() function requires a (ttf) font file to write text to an image. The basic syntax of imagettftext()function is given below:
imagettftext(image resource,font size, angle, x, y, text colour, font file, sample Text);
Let us use the above imagettftext() function to write a simple text to an image using php code given below:
<?php
header('Content-type: image/jpeg');
$sampleText = "Sample Text!";
$fontSize=30;
$imageWidth=300;
$imageHeight=100;
$image=imagecreate($imageWidth,$imageHeight);
imagecolorallocate($image,255,255,255);
$textColor=imagecolorallocate($image,0,0,0);
imagettftext($image,$fontSize,0,15,60,$textColor, 'font.ttf',$sampleText);
imagejpeg($image);
?>
The above php code will write the text ('Sample Text!') to an image file created using imagecreate() function. The width of the image is set to 300 and height is set to 100 pixels. The background colour allocated to the image using imagecolorallocate() function is white rgb(255,255,255) and text colour is black rgb(0,0,0). In the next step the parameters set above are passed into imagettftext() function to write text to the image. The output image of the above code should look something like this:
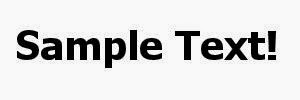
Note: Do not forget to add the font.ttf file used in the imagettftext() function above to the same directory where your php code is located. You can download TTF files here.
Playing around a bit with the parameters of imagettftext() function will produce different results.
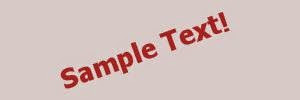
<?php
header('Content-type: image/jpeg');
$sampleText = "Sample Text!";
$fontSize=20;
$imageWidth=300;
$imageHeight=100;
$image=imagecreate($imageWidth,$imageHeight);
imagecolorallocate($image,230,219,219);
$textColor=imagecolorallocate($image,173,52,52);
imagettftext($image,$fontSize,20,65,90,$textColor, 'font.ttf',$sampleText);
imagejpeg($image);
?>
Stay tuned for more lessons...
How to create an image in PHP?
Drawing image patterns using imagesetpixel() in PHP
Convert a string to an image in PHP
Convert an image to a string in PHP
No comments:
Post a Comment