The previous lesson of lesson of PHP Lessons Series was about PHP Switch Statement and in this lesson we will learn about PHP Array Functions.
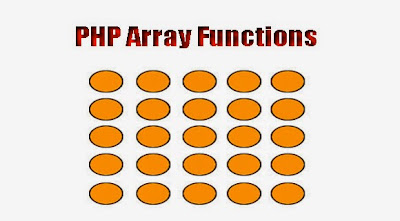
The output of the above SizeOf() function is 5, because there are 5 countries in the in the above array ($Countries) passed in the sizeof() function.
The above shuffle function will change the indexes of all the countries in the array ($Countries) randomly.
The above array_unique() function will remove the duplicate countries 'USA' and 'Italy' from the array ($Countries).
The above array ($Countries) contains countries as keys. array_key_exists() function used in above code is used to find 'Italy' as a key in the array ($Countries). As 'Italy' exists as a key in the above array so the above array_key_exists() function will return 1.
The above in_array() function is used to find the value (3) in the array ($Countries). As the value (3) exists in the above array, so the output of the above in_array() function will be 1.
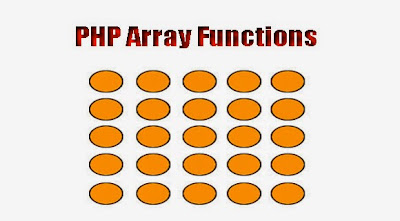
SizeOf()
Array SizeOf() function is used to count the number of elements of an array and then return the count value. To find the count of array elements, just pass the array in the sizeof() function. An example usage of sizeof() function is given below:
$Countries = array('USA','England','Italy','Germany','France');
$count = SizeOf($Countries);
echo $count;
The output of the above SizeOf() function is 5, because there are 5 countries in the in the above array ($Countries) passed in the sizeof() function.
Count()
Just like the SizeOf() function discussed above, array Count() function is also used to count the number of elements of an array. The usage of both functions is exactly same.Shuffle()
Array Shuffle() function is used to change the indexes of all the array elements randomly each time the Shuffle() function is called. To shuffle the elements of an array, just pass the array in the shuffle function. An example usage of Shuffle() function is given below:
$Countries = array('USA','England','Italy','Germany','France');
Shuffle($Countries);
print_r($Countries);
The above shuffle function will change the indexes of all the countries in the array ($Countries) randomly.
array_unique()
array_unique() function is used to remove the duplicate values from an array. To remove the duplicate values from an array, pass the array in the array_unique() function. An example usage of array_unique() function is given below:
$Countries = array('USA','England','Italy','Germany','France','USA','Italy');
$Countries = array_unique($Countries);
print_r($Countries);
The above array_unique() function will remove the duplicate countries 'USA' and 'Italy' from the array ($Countries).
array_key_exists()
array_key_exists() function is used to find out if a specific key exists in an array. For proper functionality just pass the (key to find in the array and the array) in the array_key_exists() function. An example usage of array_key_exists() function is given below:
$Countries = array('USA' => '1','England' => '2','Italy' => '3',
'Germany' => '4','France' => '5');
$result = array_key_exists('Italy',$Countries);
echo $result;
The above array ($Countries) contains countries as keys. array_key_exists() function used in above code is used to find 'Italy' as a key in the array ($Countries). As 'Italy' exists as a key in the above array so the above array_key_exists() function will return 1.
in_array()
in_array() function is used to find if a specific value exists in an array. For proper functionality just pass the (value to find in the array and the array) in the in_array() function. An example usage of in_array() function is given below:
$Countries = array('USA' => '1','England' => '2','Italy' => '3',
'Germany' => '4','France' => '5');
$result = in_array('3',$Countries);
echo $result;
The above in_array() function is used to find the value (3) in the array ($Countries). As the value (3) exists in the above array, so the output of the above in_array() function will be 1.
No comments:
Post a Comment