The previous lesson of PHP Lessons Series was about PHP Array Functions and in this
lesson we will learn about PHP Array Sorting Functions.
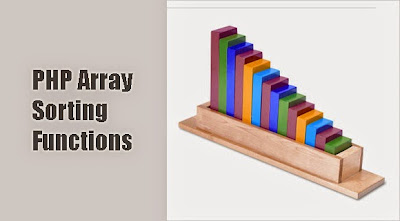
If we apply sort() function on the above array, the countries will be sorted in ascending order alphabetically. An example of array sort function applied on an alphabetic array is given below:
Similarly if we have a numeric array ($Numbers) given below:
And we apply sort() function on it, the numbers in the array ($Numbers) will be sorted in ascending order. An example of array sort function applied on a numeric array is given below:
If we have an array containing both numeric and alphabetic values as array elements i.e
And we apply sort function on it, then first the numeric values will be sorted and then the alphabetic values. An example of array sort function applied on a mixed array is given below:
If we apply asort() function on the above array, the cities (values) against the countries (keys) will be sorted in ascending order alphabetically. An example of asort() function is given below:
If we apply ksort() function on the above array, the countries (keys) against the cities (values) will be sorted in ascending order alphabetically. An example of ksort() function is given below:
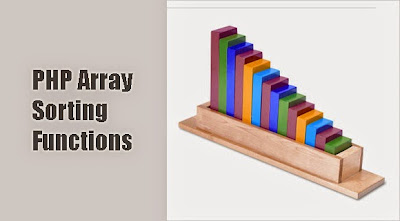
sort()
PHP sort() function is used to sort the elements of an array in ascending order. Consider the array ($Countries) given below:
$Countries = array('USA','England','Germany','France');
If we apply sort() function on the above array, the countries will be sorted in ascending order alphabetically. An example of array sort function applied on an alphabetic array is given below:
$Countries = array('USA','England','Germany','France');
sort($Countries);
print_r($Countries);
Similarly if we have a numeric array ($Numbers) given below:
$Numbers = array('155','21','12','65');
And we apply sort() function on it, the numbers in the array ($Numbers) will be sorted in ascending order. An example of array sort function applied on a numeric array is given below:
$Numbers = array('155','21','12','65');
sort($Numbers);
print_r($Numbers);
If we have an array containing both numeric and alphabetic values as array elements i.e
$MixedArray = array('155','USA','Italy','65');
And we apply sort function on it, then first the numeric values will be sorted and then the alphabetic values. An example of array sort function applied on a mixed array is given below:
$MixedArray = array('155','USA','Italy','65');
sort($MixedArray);
print_r($MixedArray);
rsort()
PHP rsort() function is used to sort the elements of an array in descending order. The functionality of rsort() function is exactly same as sort() function but the only difference between the two is that sort() function sorts the array elements in ascending order while rsort() function sorts the array elements in descending order.asort()
PHP asort() function is used to sort an associative array in ascending order according to value. The sort() function discussed above could not sort an associative array. Consider the associative array ($Countries) given below:
$Countries = array('USA'=>'Washington DC','England'=>'London',
'Germany'=>'Berlin','France'=>'Paris');
If we apply asort() function on the above array, the cities (values) against the countries (keys) will be sorted in ascending order alphabetically. An example of asort() function is given below:
$Countries = array('USA'=>'Washington DC','England'=>'London',
'Germany'=>'Berlin','France'=>'Paris');
asort($Countries);
print_r($Countries);
arsort()
PHP arsort() function is used to sort an associative array in descending order according to value. The functionality of arsort() function is exactly same as asort() function but the only difference between the two is that asort() function sorts the values of an associative array in ascending order while arsort() function sorts the values of an associative array in descending order.ksort()
PHP ksort() function is used to sort an associative array in ascending order according to key. Consider the associative array ($Countries) given below:
$Countries = array('USA'=>'Washington DC','England'=>'London',
'Germany'=>'Berlin','France'=>'Paris');
If we apply ksort() function on the above array, the countries (keys) against the cities (values) will be sorted in ascending order alphabetically. An example of ksort() function is given below:
$Countries = array('USA'=>'Washington DC','England'=>'London',
'Germany'=>'Berlin','France'=>'Paris');
ksort($Countries);
print_r($Countries);
No comments:
Post a Comment