The previous lesson of PHP Lessons Series was about PHP String Conversion Functions and in this lesson we will learn about Switch Statement in PHP.
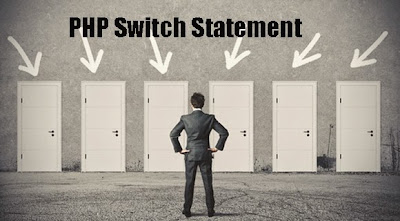
The basic structure of a switch statement is given below:
It is clear from the above structure of the switch statement that a variable value is passed in the switch statement and the value of each case is checked with the value passed in the switch to decide which case to execute. If the value of a case matches the value passed in the switch, the code inside the case is executed and then the break statement breaks out of the switch statement so no more cases are checked. If the value passed in the switch does not match the values of any case, then the default case will run and the code inside it will be executed. An example code of a switch statement is given below:
In the above example we have passed a variable $n in the switch statement and it's value is set equal to 3. As the value passed in the switch matches the value of case 3, so the code inside it will be executed and will echo "3rd condition is executed". If the value of $n is set to 5 then it will not match the value of any case so the default condition of case will run for $n = 5.
If there is no break in the case statements and the value passed in the switch matches the value of a case, then the code inside the case will be executed and as there is no break after the code so the switch will not break and the code inside all the succeeding cases will execute including that of the default case. An example code of the situation is given below:
In the above code the value of $n matches case 3, so the code inside case 3 will be executed and after that the switch will not break and the code inside case 4 and default will also execute. The output of the above code is given below:
3rd condition is executed
4th condition is executed
Default condition is executed
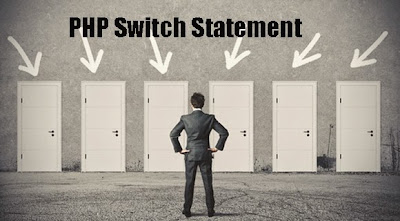
The basic structure of a switch statement is given below:
switch(variable){
case value1:
// code to be executed
break;
case value2:
// code to be executed
break;
case value3:
// code to be executed
break;
case value4:
// code to be executed
break;
case default:
// code to be executed
}
It is clear from the above structure of the switch statement that a variable value is passed in the switch statement and the value of each case is checked with the value passed in the switch to decide which case to execute. If the value of a case matches the value passed in the switch, the code inside the case is executed and then the break statement breaks out of the switch statement so no more cases are checked. If the value passed in the switch does not match the values of any case, then the default case will run and the code inside it will be executed. An example code of a switch statement is given below:
$n = 3;
switch($n){
case 1:
echo '1st condition is executed<br />';
break;
case 2:
echo '2nd condition is executed<br />';
break;
case 3:
echo '3rd condition is executed<br />';
break;
case 4:
echo '4th condition is executed<br />';
break;
default:
echo 'Default condition is executed';
}
In the above example we have passed a variable $n in the switch statement and it's value is set equal to 3. As the value passed in the switch matches the value of case 3, so the code inside it will be executed and will echo "3rd condition is executed". If the value of $n is set to 5 then it will not match the value of any case so the default condition of case will run for $n = 5.
If there is no break in the case statements and the value passed in the switch matches the value of a case, then the code inside the case will be executed and as there is no break after the code so the switch will not break and the code inside all the succeeding cases will execute including that of the default case. An example code of the situation is given below:
$n = 3;
switch($n){
case 1:
echo '1st condition is executed<br />';
case 2:
echo '2nd condition is executed<br />';
case 3:
echo '3rd condition is executed<br />';
case 4:
echo '4th condition is executed<br />';
default:
echo 'Default condition is executed';
}
In the above code the value of $n matches case 3, so the code inside case 3 will be executed and after that the switch will not break and the code inside case 4 and default will also execute. The output of the above code is given below:
3rd condition is executed
4th condition is executed
Default condition is executed
No comments:
Post a Comment