The previous lesson of PHP Lessons Series was about How to remove backslashes in PHP and in this lesson we will learn to use Functions in PHP.
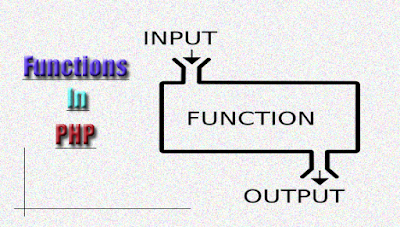
The use of functions is very important in any programming language, as it empowers us with the reusability of a certain code. We create a function, write a certain code in it and then call the function to run the code inside the function.
You can see in the above example code the function call is made before creating the function but it's ok if you write the function before or after the function call, as long as the function is present it will work fine.
In the above addition function, addition operation of two variables is performed and then the result is stored in a third variable which is returned by the function. In the function call the variable ($result) receives the value returned by the addition function. You can echo the result of the above addition function like this:
Or
The above code shows the values ($val1 and $val2) passed in the addition function. In addition function the values are received in variables ($a and $b), addition operation is performed and result ($c) is returned by the addition function.
The above factorial calculator function will calculate the factorial of the number passed in it. Just play with the value of variable ($number) for different factorial results.
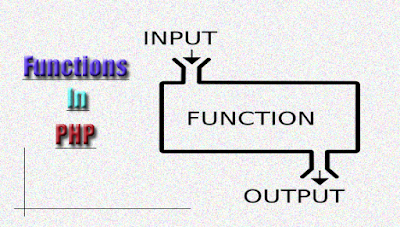
The use of functions is very important in any programming language, as it empowers us with the reusability of a certain code. We create a function, write a certain code in it and then call the function to run the code inside the function.
How to create a function in PHP?
To create a PHP function, write the keyword "function" followed by the name of the function and then the opening and closing curly brackets containing the function code. The basic structure of a PHP function is given below:
function Name(){
// Code to executed here
}
How to call a function in PHP?
Calling a PHP function is very simple, all you have to do to call the above created Name() function in your code is write the code given below:
Name();
Example code of function creation and call
Let us create and a simple function that echos 'Hello World!' i.e
helloworld();
function helloworld(){
echo 'Hello World!';
}
You can see in the above example code the function call is made before creating the function but it's ok if you write the function before or after the function call, as long as the function is present it will work fine.
How to return a function value in PHP?
We can also perform certain calculations in a function and return the result of the calculations like this:
$result = addition();
function addition(){
$a = 10, $b = 20;
$c = $a + $b;
return $c;
}
In the above addition function, addition operation of two variables is performed and then the result is stored in a third variable which is returned by the function. In the function call the variable ($result) receives the value returned by the addition function. You can echo the result of the above addition function like this:
echo 'The result is '.addition();
Or
echo 'The result is '.addition();
How to pass values in a PHP function?
The variables used in the above addition function were initialized inside the function. If we want to pass the values to be added from outside the function, this is how we can do it:
$val1 = 10, $val2 = 20;
$result = addition($val1,$val2);
function addition($a, $b){
$c = $a + $b;
return $c;
}
The above code shows the values ($val1 and $val2) passed in the addition function. In addition function the values are received in variables ($a and $b), addition operation is performed and result ($c) is returned by the addition function.
Example factorial calculation function in PHP
$number = 10;
$Factorial = CalculateFactorial($number);
echo $Factorial;
function CalculateFactorial($num){
$result = $num;
for($i=$num-1;$i>=1;$i--){
$result = $result * $i;
}
return $result;
}
The above factorial calculator function will calculate the factorial of the number passed in it. Just play with the value of variable ($number) for different factorial results.
No comments:
Post a Comment