The previous lesson of javascript lessons series was about Javascript Load Events and in this lesson we will learn to create html elements in javascript.
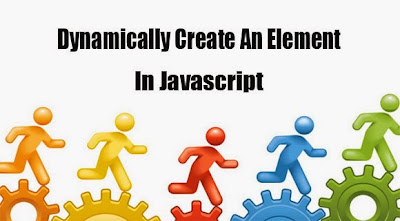
We can dynamically create an html element using document.createElement() in javascript. The basic syntax of document.createElement() is given below:
Document.createElement() creates an html element but it won't show in the html document until we append it to some parent html element. Let us dynamically create a div element in javascript and then append it to the html document body. Example code is given below:
HTML Code:
Javascript Code:
The above javascript code will create a div element, assign some attributes values to the div and finally append it to the body of html document. Check the demo of dynamically creating and appending divs to the container div given below:
The html, css and javascript code is given below to create the above demo yourself.
HTML Code:
Css Code:
Javascript Code:
For more lessons on Javascript subscribe on InformationBitz.com
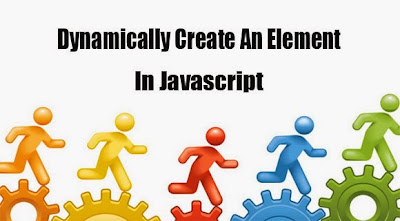
We can dynamically create an html element using document.createElement() in javascript. The basic syntax of document.createElement() is given below:
var element = document.createElement(tagName);
Document.createElement() creates an html element but it won't show in the html document until we append it to some parent html element. Let us dynamically create a div element in javascript and then append it to the html document body. Example code is given below:
HTML Code:
<body></body>
Javascript Code:
<script type="text/javascript">
var div = document.createElement("div");
div.style.width = "200px";
div.style.height = "80px";
div.style.background = "red";
document.body.appendChild(div);
</script>
The above javascript code will create a div element, assign some attributes values to the div and finally append it to the body of html document. Check the demo of dynamically creating and appending divs to the container div given below:
Demo : Dynamically create div in javascript
Create divs dynamically.
Create divs dynamically.
The html, css and javascript code is given below to create the above demo yourself.
HTML Code:
<b style="color:red;font-weight:normal;line-height:75px;">Create
<select id="numberofballs">
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select> divs dynamically.
</b>
<input type="button" value="Create!" id="creatediv"
onclick="creatediv();"/>
<div class="demoContainer" id="demoContainer"></div>
Css Code:
.demo{
padding-top:15px;
padding-left:10px;
padding-right:10px;
font-size:14px;
font-family:arial;
font-weight:normal;
margin-bottom:10px;
background:#FFFFFF;
}
.demoContainer{
border-radius:10px;
border:3px solid blue;
min-height:100px;
width:300px;
overflow:hidden;
padding:10px;
}
.demoBall{
width:20px;
height:20px;
background:red;
border:1px solid #000000;
border-radius:15px;
float:left;
}
Javascript Code:
<script type="text/javascript">
function creatediv(){
var numberofballs = document.getElementById("numberofballs").value;
var demoContainer = document.getElementById("demoContainer");
for(var i=0;i < numberofballs;i++){
var div = document.createElement("div");
div.className = "demoBall";
demoContainer.appendChild(div);
}
}
</script>
For more lessons on Javascript subscribe on InformationBitz.com
No comments:
Post a Comment