The previous lesson of PHP Lessons Series was about selecting data from mysql database in php and in this lesson we will learn to force a file download in php using readfile() function and CURL separately.
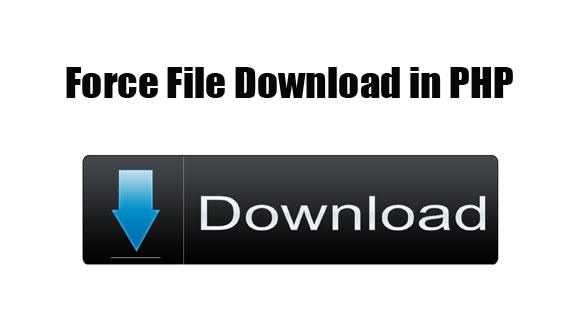
An easy way to force the image download when someone clicks on the above link is by redirecting the user to download.php file and pass the parameter download_file_name in the url. The php code of the file (download.php) will do the rest to download the image with the file name passed in the url.
PHP file download.php
The above php code will download image.png file.
The link will remain the same, we need to change the php code inside the file (download.php). The PHP code of file download using CURL is given below:
PHP file download.php
The above php code will copy the file image.png from some other server to tmp.png created on your server.
Stay tuned for more lessons...
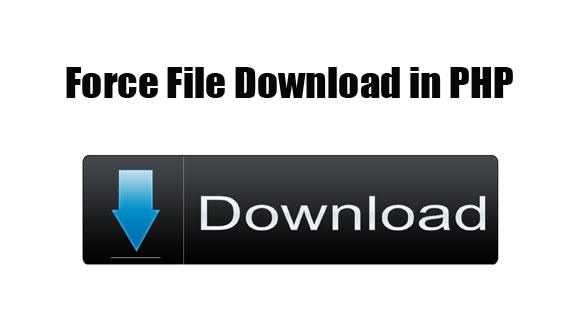
PHP file download using readfile() function:
Let us say we have a text link (Download File) and we want to download a png image when someone clicks on the link. Consider the text link given below:
<a href="download.php?download_file_name=image.png">Download File</a>
An easy way to force the image download when someone clicks on the above link is by redirecting the user to download.php file and pass the parameter download_file_name in the url. The php code of the file (download.php) will do the rest to download the image with the file name passed in the url.
PHP file download.php
<?php
if(isset($_GET['download_file_name'])){
$file = $_GET['download_file_name']; // get the file name
$file = str_replace(" ","%20",$file); // replace the spaces in the file name with %20
if(file_exists($file)){
header('Content-Description: File Transfer');
header('Content-Type: application/octet-stream');
header('Content-Disposition: attachment; filename='.basename($file));
header('Expires: 0');
header('Cache-Control: must-revalidate');
header('Pragma: public');
header('Content-Length: '.filesize($file));
readfile($file);
exit;
}
}
?>
The above php code will download image.png file.
PHP file download using CURL:
Just like in the case of readfile() function, we will use the text image link to download the image i.e
<a href="download.php?download_file_name=image.png">Download File</a>
The link will remain the same, we need to change the php code inside the file (download.php). The PHP code of file download using CURL is given below:
PHP file download.php
<?php
if(isset($_GET['download_file_name'])){
$file = $_GET['download_file_name'];
$file = str_replace(" ","%20",$file);
if(file_exists($file)){
set_time_limit(0);
// create and open a temp png file to save the downloaded file
$fileopen = fopen ('tmp.png', 'w+');
if($fileopen){
$ch = curl_init($file);
curl_setopt($ch, CURLOPT_URL, $file);
curl_setopt($ch, CURLOPT_FILE, $fileopen);
curl_setopt($ch, CURLOPT_HEADER, 0);
curl_setopt($ch, CURLOPT_TIMEOUT, 50); // set time out
$data = curl_exec($ch); // curl execute
if ($data === FALSE) { // if curl execution fails
// show the curl execution error
die("Curl failed: " . curL_error($ch));
}
curl_close($ch);
fclose($fileopen);
}else{
echo 'File is not opened';
}
}
}
?>
The above php code will copy the file image.png from some other server to tmp.png created on your server.
Stay tuned for more lessons...
No comments:
Post a Comment