The previous lesson of PHP Lessons Series was about PHP Loops and in this lesson we will learn about Multidimensional Arrays in PHP.
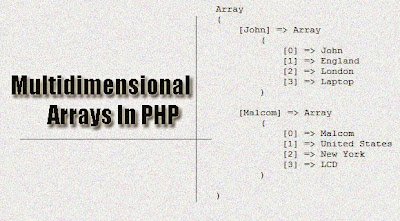
There are two types of multidimensional arrays in php:
1. Multidimensional Indexed Arrays
2. Multidimensional Associative Arrays
The above code shows a multidimensional indexed array ($multidimentional_indexed_array). The 0th index of $multidimentional_indexed_array will contain the details of John and the first index will contain the details of Malcom. Another method of initializing a multidimensional indexed array is given below:
The above code shows the use of a for loop to echo the values of the above multidimensional array.
The above code shows the use of a foreach loop to echo the values of the above multidimensional array.
In the above example of multidimensional indexed arrays, we had an associative array at every index of the main indexed array. We can also have an indexed array at every index of the main indexed array i.e
Another method of initialization is given below:
The above code shows the use of a for loop to echo the values of the above multidimensional array.
The above code shows the use of a foreach loop to echo the values of the above multidimensional array.
The above code shows a multidimensional associative array ($multidimensional_associative_array). The key (John) of $multidimensional_associative_array has an array as a value which contain the details of John and the key (Malcom) has an array as a value which contain the details of Malcom. Another method of initializing a multidimensional associative array is given below:
The above code shows the use of a foreach loop to echo the values of the above multidimensional array.
In the above example of multidimensional associative arrays, we had an associative array as value in every key value pair of the main associative array. We can also have an indexed array as value in each key value pair of the main associative array i.e
Another method of initialization is given below:
The above code shows the use of a foreach loop to echo the values of the above multidimensional array.
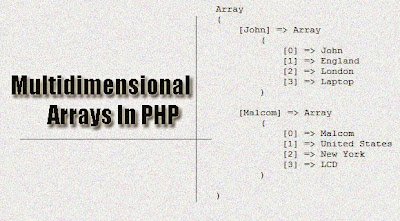
There are two types of multidimensional arrays in php:
1. Multidimensional Indexed Arrays
2. Multidimensional Associative Arrays
Multidimensional Indexed Arrays:
A multidimensional indexed array contains an array at every index of the main array i.e
$multidimensional_indexed_array=array(array('Name'=>'John',
'Country'=>'England',
'City'=>'London',
'Product'=>'Laptop'),
array('Name'=>'Malcom',
'Country'=>'United States',
'City'=>'New York',
'Product'=>'LCD'));
The above code shows a multidimensional indexed array ($multidimentional_indexed_array). The 0th index of $multidimentional_indexed_array will contain the details of John and the first index will contain the details of Malcom. Another method of initializing a multidimensional indexed array is given below:
$multidimensional_indexed_array[0] = array('Name'=>'John',
'Country'=>'England',
'City'=>'London',
'Product'=>'Laptop');
$multidimensional_indexed_array[1] = array('Name'=>'Malcom',
'Country'=>'United States',
'City'=>'New York',
'Product'=>'LCD');
Using a for loop to echo the values of the above array:
for ($i=0;$i<2;$i++)
{
echo '<br />The name is '.
$multidimensional_indexed_array[$i]['Name'].
' and the country is '.
$multidimensional_indexed_array[$i]['Country'];
}
The above code shows the use of a for loop to echo the values of the above multidimensional array.
Using a foreach loop to echo the values of the above array:
foreach ($multidimensional_indexed_array as $key => $value)
{
echo '<br />The name is '.
$value['Name'].
' and the country is '.
$value['Country'];
}
The above code shows the use of a foreach loop to echo the values of the above multidimensional array.
In the above example of multidimensional indexed arrays, we had an associative array at every index of the main indexed array. We can also have an indexed array at every index of the main indexed array i.e
$multidimensional_indexed_array=array(array('John',
'England',
'London',
'Laptop'),
array('Malcom',
'United States',
'New York',
'LCD'));
Another method of initialization is given below:
$multidimensional_indexed_array[0] = array('John',
'England',
'London',
'Laptop');
$multidimensional_indexed_array[1] = array('Malcom',
'United States',
'New York',
'LCD');
Using a for loop to echo the values of the above array:
for ($i=0;$i<2;$i++)
{
echo '<br />The name is '.
$multidimensional_indexed_array[$i][0].
' and the country is '.
$multidimensional_indexed_array[$i][1];
}
The above code shows the use of a for loop to echo the values of the above multidimensional array.
Using a foreach loop to echo the values of the above array:
foreach ($multidimensional_indexed_array as $key => $value)
{
echo '<br />The name is '.$value[0].' and the country is '.$value[1];
}
The above code shows the use of a foreach loop to echo the values of the above multidimensional array.
Multidimensional Associative Arrays:
A multidimensional associative array contains an array as value against each key in the key value pair of the main associative array i.e
$multidimensional_associative_array=array(
'John'=>array('Name'=>'John',
'Country'=>'England',
'City'=>'London',
'Product'=>'Laptop'),
'Malcom'=>array('Name'=>'Malcom',
'Country'=>'United States',
'City'=>'New York',
'Product'=>'LCD'));
The above code shows a multidimensional associative array ($multidimensional_associative_array). The key (John) of $multidimensional_associative_array has an array as a value which contain the details of John and the key (Malcom) has an array as a value which contain the details of Malcom. Another method of initializing a multidimensional associative array is given below:
$multidimensional_associative_array['John'] = array('Name'=>'John',
'Country'=>'England',
'City'=>'London',
'Product'=>'Laptop');
$multidimensional_associative_array['Malcom'] = array('Name'=>'Malcom',
'Country'=>'United States',
'City'=>'New York',
'Product'=>'LCD');
Using a foreach loop to echo the values of the above array:
foreach ($multidimensional_associative_array as $key => $value)
{
echo '<br />The name is '.
$value['Name'].
' and the country is '.
$value['Country'];
}
The above code shows the use of a foreach loop to echo the values of the above multidimensional array.
In the above example of multidimensional associative arrays, we had an associative array as value in every key value pair of the main associative array. We can also have an indexed array as value in each key value pair of the main associative array i.e
$multidimensional_associative_array=array('John'=>array('John',
'England',
'London',
'Laptop'),
'Malcom'=>array('Malcom',
'United States',
'New York',
'LCD'));
Another method of initialization is given below:
$multidimensional_associative_array['John'] = array('John',
'England',
'London',
'Laptop');
$multidimensional_associative_array['Malcom'] = array('Malcom',
'United States',
'New York',
'LCD');
Using a foreach loop to echo the values of the above array:
foreach ($multidimensional_associative_array as $key => $value)
{
echo '<br />The name is '.$value[0].' and the country is '.$value[1];
}
The above code shows the use of a foreach loop to echo the values of the above multidimensional array.
No comments:
Post a Comment