In this lesson we will learn to fill an html5 canvas with colors. Basically we will use onmousemove event to draw a circle at
the current co-ordinates of mouse on canvas. So, circles of a particular color are drawn at the new co-ordinates of canvas as the mouse moves on canvas.
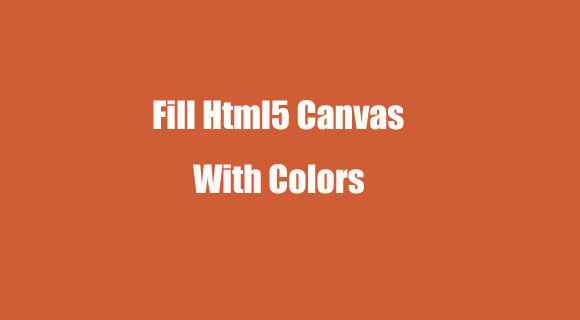
Demo: Fill Html5 Canvas with Colors
Html Code:
In the above html code onmousemove event is bind to the html5 canvas. Javascript fill() function will be called each time the mouse co-ordiantes changes on canvas.
Css Code:
Javascript Code:
Stay tuned for more lessons...
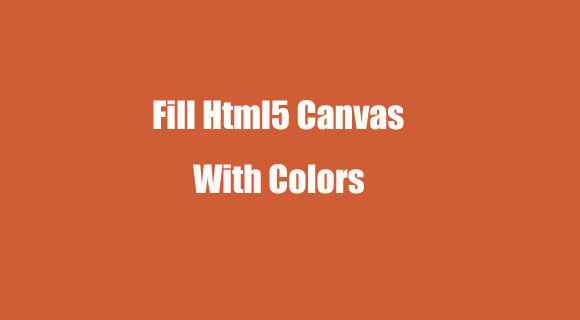
Demo: Fill Html5 Canvas with Colors
Html Code:
<div class="options">
<div class="colors">
<div class="box"><div class="rect" id="red" onclick="setColor('red');"
style="background:red;"></div></div>
<div class="box"><div class="rect" id="green" onclick="setColor('green');"
style="background:green;"></div></div>
<div class="box"><div class="rect" id="blue" onclick="setColor('blue');"
style="background:blue;"></div></div>
<div class="box"><div class="rect" id="orange" onclick="setColor('orange');"
style="background:orange;"></div></div>
<div class="box"><div class="rect" id="yellow" onclick="setColor('yellow');"
style="background:yellow;"></div></div>
<div class="box"><div class="rect" id="pink" onclick="setColor('pink');"
style="background:pink;"></div></div>
<div class="box"><div class="rect" id="black" onclick="setColor('black');"
style="background:black;"></div></div>
<div class="box"><div class="rect" id="brown" onclick="setColor('brown');"
style="background:brown;"></div></div>
</div>
</div>
<canvas id="paint" width="600" height="350" onmousemove="fill(event);"></canvas>
In the above html code onmousemove event is bind to the html5 canvas. Javascript fill() function will be called each time the mouse co-ordiantes changes on canvas.
Css Code:
<style>
canvas{
border:1px solid black;
}
.box{
padding:2px;
border:2px solid #000000;
width:15px;
float:left;
margin-left:2px;
}
.rect{
width:10px;
height:10px;
border:1px solid #000000;
}
.circ{
width:10px;
height:10px;
border-radius:20px;
border:1px solid #000000;
}
.colors{
width:52px;
}
</style>
Javascript Code:
<script type="text/javascript">
var color = 'white';
function setColor(n){
color = n;
var r = document.getElementById('red');
var g = document.getElementById('green');
var b = document.getElementById('blue');
var o = document.getElementById('orange');
var y = document.getElementById('yellow');
var p = document.getElementById('pink');
var bl = document.getElementById('black');
var br = document.getElementById('brown');
r.style.opacity = "1";
g.style.opacity = "1";
b.style.opacity = "1";
o.style.opacity = "1";
y.style.opacity = "1";
p.style.opacity = "1";
bl.style.opacity = "1";
br.style.opacity = "1";
var colorid = document.getElementById(color);
colorid.style.opacity = "0.4";
}
function fill(e){
if (e == null)
e = window.event;
if (e.x != undefined && e.y != undefined)
{
x = e.x;
y = e.y;
}
else // Firefox method to get the position of mouse
{
x = e.clientX + document.body.scrollLeft +
document.documentElement.scrollLeft;
y = e.clientY + document.body.scrollTop +
document.documentElement.scrollTop;
}
var paint = document.getElementById('paint');
x -= paint.offsetLeft; // x-position of mouse
y -= paint.offsetTop; // y-position of mouse
var ctx = paint.getContext('2d');
ctx.fillStyle = color;
ctx.beginPath();
ctx.arc(x,y,20,0,2*Math.PI); // Draw circle at the current position of mouse
ctx.fill();
}
</script>
Stay tuned for more lessons...
No comments:
Post a Comment