The previous lesson of PHP Lessons Series was about PHP String Functions and in this lesson we will learn about PHP String Comparison Functions.
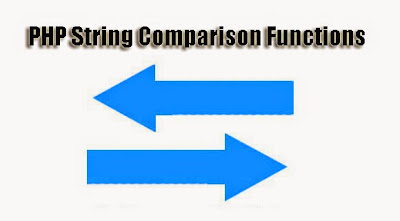
The output of the above similar_text() function will be 21, as there are 21 characters similar between the two strings. The integer value (21) returned by similar_text function will be saved in the variable $similarity.
Pass the characters to replace, characters to replace with and string in str_ireplace() function for functionality. An example of str_ireplace() is given below:
The output of the above str_ireplace() function will be "This is a modified string.", as the word "sample" in the string will be replaced with "modified". The string value ('This is a modified string.') returned by str_ireplace function will be saved in the string variable $str.
Pass a string and the character in strcspn() function for functionality. An example of strcspn() is given below:
The output of the above str_ireplace() function will be 3, as there are 3 characters before the first occurrence of 's' in the string "This is a sample string.". The integer value (3) returned by strcspn() function will be saved in the variable $val.
Pass a string and a character or a group of characters in stripos() function for functionality. An example of stripos() function is given below:
The output of the above stripos() function will be 20, as there are 20 characters before the first occurrence of characters "in" in the string "This is a sample string.". The integer value (20) returned by stripos() function will be saved in the variable $val.
Pass the strings in the strcasecmp() function for functionality. An example of strcasecmp() function is given below:
The output of the above strcasecmp() function will be -1, as the binary value of the first string is less than the binary value of the second string by 1.
Related Posts
PHP String Conversion Functions
PHP String Functions
More about PHP String Functions
How to remove backslashes in php?
How to add backslashes in php?
Explode and Implode functions in PHP
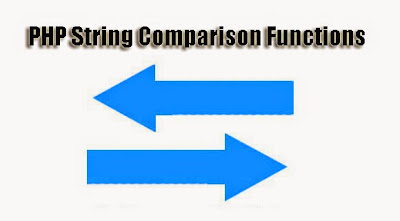
similar_text()
similar_text() function is used to count the number of characters that are similar between two strings and returns an integer value. Pass the strings in the similar_text() function to find the similarity between the two strings. The usage of similar_text() function is given below:
$similarity = similar_text("This is a test string","This is another test string");
The output of the above similar_text() function will be 21, as there are 21 characters similar between the two strings. The integer value (21) returned by similar_text function will be saved in the variable $similarity.
str_ireplace()
str_ireplace() function is used to replace a character or a group of characters in a string with other characters. The basic structure of str_ireplace() function is given below:
str_ireplace(characters to replace,characters to replace with,string);
Pass the characters to replace, characters to replace with and string in str_ireplace() function for functionality. An example of str_ireplace() is given below:
$str = str_ireplace("sample","modified","This is a sample string.");
The output of the above str_ireplace() function will be "This is a modified string.", as the word "sample" in the string will be replaced with "modified". The string value ('This is a modified string.') returned by str_ireplace function will be saved in the string variable $str.
strcspn()
strcspn() function returns the number of characters in a string before the first occurrence of a specific character. The basic structure of strcspn() function is given below:
strcspn(string,character);
Pass a string and the character in strcspn() function for functionality. An example of strcspn() is given below:
$val = strcspn("This is a sample string.","s");
The output of the above str_ireplace() function will be 3, as there are 3 characters before the first occurrence of 's' in the string "This is a sample string.". The integer value (3) returned by strcspn() function will be saved in the variable $val.
stripos()
stripos() function returns the number of characters in a string before the first occurrence of a single character or a group of characters specified. The basic structure of stripos() function is given below:
stripos(string,character/characters);
Pass a string and a character or a group of characters in stripos() function for functionality. An example of stripos() function is given below:
$val = stripos("This is a sample string.","in");
The output of the above stripos() function will be 20, as there are 20 characters before the first occurrence of characters "in" in the string "This is a sample string.". The integer value (20) returned by stripos() function will be saved in the variable $val.
strcasecmp()
strcasecmp() is a case-insensitive (treats the capital letters and small letters the same), binary-safe (evaluates the input data in binary form) string comparison function. It returns an integer value showing how different the two strings are. If the strings are exactly the same regardless of the letter case, the output of the strcasecmp() function will be 0. If the first string is smaller than the second string the output will be less than 0 and vice versa. The basic structure of strcasecmp() function is given below:
strcasecmp(string1,string2);
Pass the strings in the strcasecmp() function for functionality. An example of strcasecmp() function is given below:
$str_cmp = strcasecmp("This is string1","This is string2");
The output of the above strcasecmp() function will be -1, as the binary value of the first string is less than the binary value of the second string by 1.
strcmp()
The only difference between strcasecmp() and strcmp() is that unlike strcasecmp(), strcmp() is case-sensitive i.e lowercase and uppercase letters are not treated the same in strcmp().Related Posts
PHP String Conversion Functions
PHP String Functions
More about PHP String Functions
How to remove backslashes in php?
How to add backslashes in php?
Explode and Implode functions in PHP
No comments:
Post a Comment